|
CS152, Fall 2020
Computer Programming Fundamentals
Home
Schedule
Syllabus
Style Guidelines
Assignment 6: Classes, Objects, and Monsters
Due: Monday October 5th by 9:30am
Description
This is an individual assignment. Write a program that displays a grid of random silly monsters on the screen as shown in the picture below. Your program should include a "Monster" class as well as the following additional classes: Mouth, Eyes, Name. The Monster class should draw
a face with eyes (and eyebrows) and a mouth as well as a name under the face. The Monster class should have (at least) the following instance variables: xPosition, yPosition, size, monsterColor, mouth (type Mouth), eyes (type Eyes), and monsterName (type Name). The Monster class should have (at least) the following methods: constructor, displayMonster(). The Mouth, Eyes, and Name classes should include (at least) the variables and methods included in the sample code. Here is sample code for the classes you should work from. Note: you may want to add additional instance variables and/or methods to some of the classes. Here is sample code for the main program that you should work from.
The Monster constructor should create a "random" monster. That is, it should create a monster with an expression or look that is set randomly. The eyes, mouth, hair, and name must change randomly for each new monster that is created. Change more than just the color for each of these features.
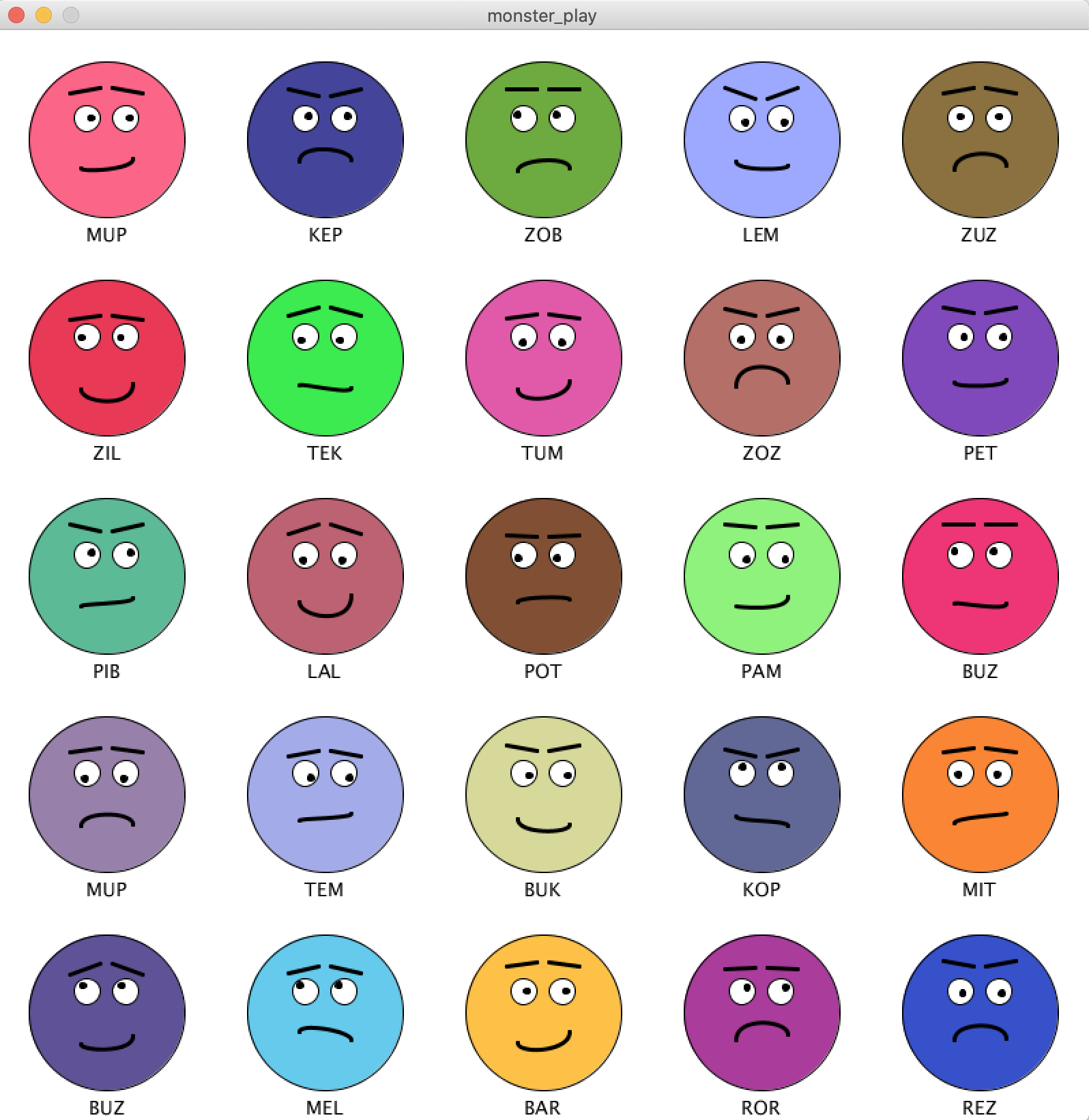
Note: chars do not work properly in repl.it. If you can work in Processing, you should. If you can only work in repl.it, you should use
arrays of strings instead of arrays of chars in your code. See this alternative repl.it sample class code.
Requirements
Your project should include all of the elements below.
- Your program should include the following classes: Monster, Mouth, Eyes, Name and follow the sample code template.
- Your program should draw a 5 x 5 grid of evenly spaced monsters on the screen, as in the picture above.
- Each monster's name should be drawn, centered, underneath it, as in the picture above.
- If the screen size is increased, the size of the monsters should increase, while remaining evenly spaced. If the screen size is decreased, the size of the monsters should decrease, while remaining evenly spaced. Your code should not assume that you will always have a square screen.
- Every monster should have (at least) eyes, eyebrows, a mouth, and a name.
- Your constructor should create a random monster. The eyes, mouth, color, and name must be randomly generated for each monster.
- Every monster's name should be a three letter word with a vowel in the middle. Good monster names include: "GAK" "YED" "PUZ". You should generate the name by choosing letters from two different character arrays, one of vowels and one of consonants. See the sample code.
- Include the appropriate source code header.
- Carefully read and follow the course Style Guidelines.
- Your monsters should be personal and fun.
- The screen should be at least 500 pixels by 500 pixels.
- Extra credit (5 points): Add at least two additional features (nose, hair, a hat, ears, teeth, horns, etc.) to your monster. These two features should also change randomly for each new monster created.
- Extra credit (5 points): Draw (at least) your eyebrows and mouth with bezier curves instead of simple shapes and lines. Use these bezier curves to make your monster extra fancy and cool!!
What to Hand in:
- Your program. Browse to the folder containing your code files. Compress the folder into a .zip file and upload this to Learn. If you do not know how to create .zip files, check out these instructions for PC and mac OS.
- A paragraph about your project. In Learn, also submit a paragraph about the game and how you designed and implemented it. Include a brief discussion about your experience working on this assignment: what challenges did you encounter? how did you address them? what was hard? what did you learn?
|